Inserting Array values from form into MySQL using PHP
The following example shows how to Store data from dynamically created table into mysql database using php. First We have to create HTML form with Product Name and Price input Fields. When user clicked '+' button new row will be added. As well as, when user clicked '-' button current row will be removed.
In this example we used two php files,
- config.php
- index.php
First We have to create products table in db_sample database.
products.sql
CREATE TABLE `products` ( `pid` int(10) unsigned NOT NULL AUTO_INCREMENT, `pname` varchar(45) NOT NULL DEFAULT '', `price` double(10,2) NOT NULL DEFAULT '0.00', PRIMARY KEY (`pid`) ) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=latin1;
config.php
<?php //MySQL Db Connection $host_name="localhost"; $user_name="root"; $password=""; $db_name="db_sample"; $con=mysqli_connect($host_name, $user_name, $password, $db_name); ?>
index.php
<?php include "config.php"; ?> <html> <head> <title>How to store data from dynamically created table into MySQL using PHP</title> <style> .table{ border-collapse:collapse; } .table th,.table td{ padding:10px; border:1px solid #ccc; } </style> </head> <body> <?php if(isset($_POST["submit"])){ $sql="insert into products (pname,price) values "; $rows=[]; for($i=0;$i<count($_POST["product"]);$i++){ $rows[]="('{$_POST["product"][$i]}','{$_POST["price"][$i]}')"; } $sql.=implode(",",$rows); if($con->query($sql)){ echo "Products Added Successfully"; }else{ echo "Added Failed!!!"; } } ?> <h3>Products Details</h3> <form method='post' action='index.php' > <table class='table'> <thead> <tr> <th>Product Name</th> <th>Price</th> <th>Add</th> <th>Remove</th> </tr> </thead> <tbody id="tbl"> <tr> <td><input type='text' name='product[]' required ></td> <td><input type='text' name='price[]' required></td> <td><input type='button' value='+' onclick='add_row()' ></td> <td><input type='button' value='-' onclick='remove_row(this)'></td> </tr> </tbody> <tfoot> <tr> <td colspan='4' style='text-align:right;'><input type='submit' name='submit' value='Save Details'></td> </tr> </tfoot> </table> </form> <script> function add_row() { var tr=document.createElement("tr"); tr.innerHTML="<td><input type='text' name='product[]' required ></td> <td><input type='text' name='price[]' required ></td> <td><input type='button' value='+' onclick='add_row()' class='btn btn-success btn-xs'></td> <td><input type='button' value='-' onclick='remove_row(this)' class='btn btn-danger btn-xs'></td>"; document.getElementById("tbl").appendChild(tr); } function remove_row(e) { var n=document.querySelector("#tbl").querySelectorAll("tr").length; if(n>1&&confirm("Are You Sure")==true){ var ele=e.parentNode.parentNode; ele.remove(); } } </script> </body> </html>
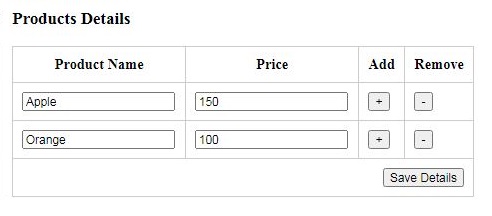