PHP MySQL Login Logout With Session
In this tutorial we will create a simple login system using the PHP and MySQL. In this example we used four php files,
- config.php
- index.php
- logout.php
- home.php
First we have to create MySQL database and table. Create database with name 'db_sample' and table name is 'users'.
Step-1 : Create 'db_sample' Database in phpmyadmin.
Open phpmyadmin page,click 'Databases' tab and enter the database name in 'Create database' field and click create button.

Step-2 : Create 'users' table in 'db_sample' database.
After database created,we have to create users table.Select 'db_sample' database from left side navbar, enter table name in 'Name' field and enter 3 in 'Number of columns' Field and click Go button.

After clicking 'Go' button, it will show the table description, like image shown in below. We have to enter column name,data type and length. Next select primary index and tick A_I button then click save button.

MySQL query for create Table
CREATE TABLE `db_sample`.`users` ( `uid` int(11) NOT NULL AUTO_INCREMENT, `uname` varchar(150) NOT NULL, `upass` varchar(150) NOT NULL, PRIMARY KEY (`uid`) ) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=latin1;
Step-3 : Add Sample User Name and Password
After creating the users table, we have to add sample user details for login. Select insert tab and enter 'admin' in uname field and '123' in upass field then click 'Go' button.
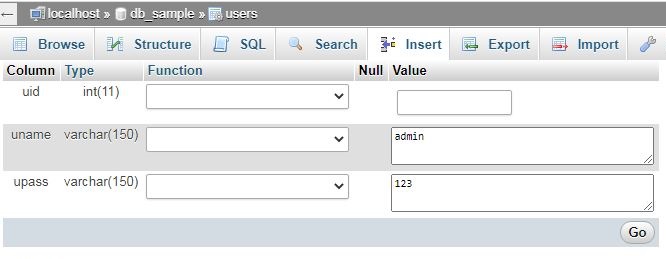
config.php
<?php //MySQL Db Connection $host_name="localhost"; $user_name="root"; $password=""; $db_name="db_sample"; $con=mysqli_connect($host_name, $user_name, $password, $db_name); ?>
index.php
<?php session_start(); include "config.php"; ?> <html> <head> <title>PHP MySql Login Logout with Session</title> <link rel='stylesheet' type='text/css' href='style.css' > </head> <body> <form action='<?php echo $_SERVER["REQUEST_URI"]; ?>' method='post' id='form'> <table> <tr> <td colspan='2' class='heading'>Login Form</td> </tr> <tr> <td><label>User Name</label></td> <td><input type='text' name='user_name' class='input' required></td> </tr> <tr> <td><label>Password</label></td> <td><input type='password' name='user_pass' class='input' required></td> </tr> <tr> <td></td> <td><input type='submit' name='submit' class='btn' value='Login'></td> </tr> </table> <?php if(isset($_POST["submit"])){ $u_name=mysqli_real_escape_string($con,$_POST["user_name"]); $u_pass=mysqli_real_escape_string($con,$_POST["user_pass"]); $sql="select uid,uname from users where uname='{$u_name}' and upass='{$u_pass}'"; $res=$con->query($sql); if($res->num_rows>0){ $row=$res->fetch_assoc(); $_SESSION["user_id"]=$row["uid"]; $_SESSION["user_name"]=$row["uname"]; header("location:home.php"); }else{ echo "<div class='msg-danger'>Invalid Login!!!</div>"; } } ?> </form> </body> </html>
logout.php
<?php session_start(); unset($_SESSION["user_id"]); unset($_SESSION["user_name"]); session_destroy(); header("location:index.php"); ?>
home.php
<?php session_start(); if(!isset($_SESSION["user_id"])){ header("location:index.php"); } ?> <html> <head> <title>PHP MySql Login Logout with Session</title> <link rel='stylesheet' type='text/css' href='style.css' > </head> <body> <div style='padding:50px;'> <p ><a href='logout.php' style='font-size:18px;'>Logout</a></p> <div class='msg-success'><b>Welcome </b> : <?php echo $_SESSION["user_name"]; ?></div> </div> </body> </html>
style.css
html,body{ margin:0 auto; } #form{ width:350px; margin:50px auto; border:1px solid #ccc; padding:5px; } #form .heading{ text-align:center; font-size:20px; font-weight:bold; } #form table td{ padding:10px 20px; } .input{ border:none; border:1px solid #ccc; padding:5px 3px; border-radius:3px; } label{ color:#33b1cc; font-weight:bold; } .btn{ border:none; border:1px solid #44ac35; background:#44ac35; color:white; padding:7px 10px; border-radius:5px; font-size:14px; } .btn:hover{ background:#36882b; } .msg-success{ width:100%; box-sizing:border-box; padding:15px 10px; background:#b3e4ab; border-radius:5px; } .msg-danger{ width:100%; box-sizing:border-box; padding:15px 10px; background:#ffc9ae; border-radius:5px; }
Final Output

