What is jQuery?
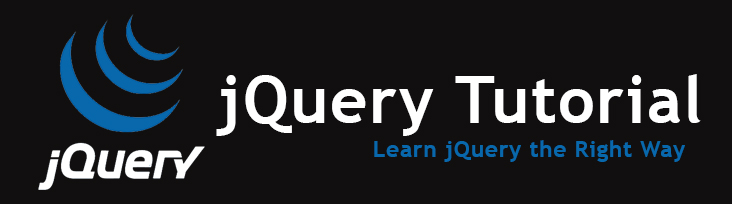
jQuery an open source JavaScript library. jQuery simplifies JavaScript programming.It's used to event handling, CSS animation and Ajax.It is a fast, small, and rich JavaScript library.The main advantage of using jQuery is that it is more easy to use and easy to learn.
How to use?
First we include jQuery library file in the head tag.Then we can written script anywhere in the body of the HTML page.
Example
<html> <head> <title>jQuery Example</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script> </head> <body> <h1></h1> <script> $(document).ready(function(){ $("h1").text("Welcome to vrsofttech"); }); </script> </body> </html>Try it Yourself
Example : Add two Numbers using jQuery
<html> <head> <title>Simple Example using jQuery</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script> </head> <body> <h4>Add Two Numbers</h4> <p> Enter Number 1 : <input type='number' id='num1'> </p> <p> Enter Number 2 : <input type='number' id='num2'> </p> <input type='button' value='Click' id='btn'> <p id='result'></p> <script> $(document).ready(function(){ $("#btn").click(function(){ let a=Number($("#num1").val()); let b=Number($("#num2").val()); let c=a+b; $("#result").text("Total : "+c); }); }); </script> </body> </html>Try it Yourself
Output
