Login with Google Account in PHP
In this tutorial, we use the Google OAuth API which is an easy and powerful way to add Google login to website.The following example shows, how to add Google Login in website using Google OAuth API with PHP.
Steps to Integrate Login with Google Account using PHP
- Create Google API project
- Download Google PHP API Client Library
- Create Google Config PHP file
- Create a Login Page,Home Page and Logout Page
Steps to Create Google API credentials
1. Go to https://console.developers.google.com. This link will open up Google Cloud Platform Dashboard
2. Click on the Select a project Dropdown. It will open up a popup as shown on image below

Click on the New Project link and it will ask you to enter the Project Name as shown on image below

Click on the Create button to save new project. It will be redirected to the Dashboard and Select Your new project from dropdown as shown on image below
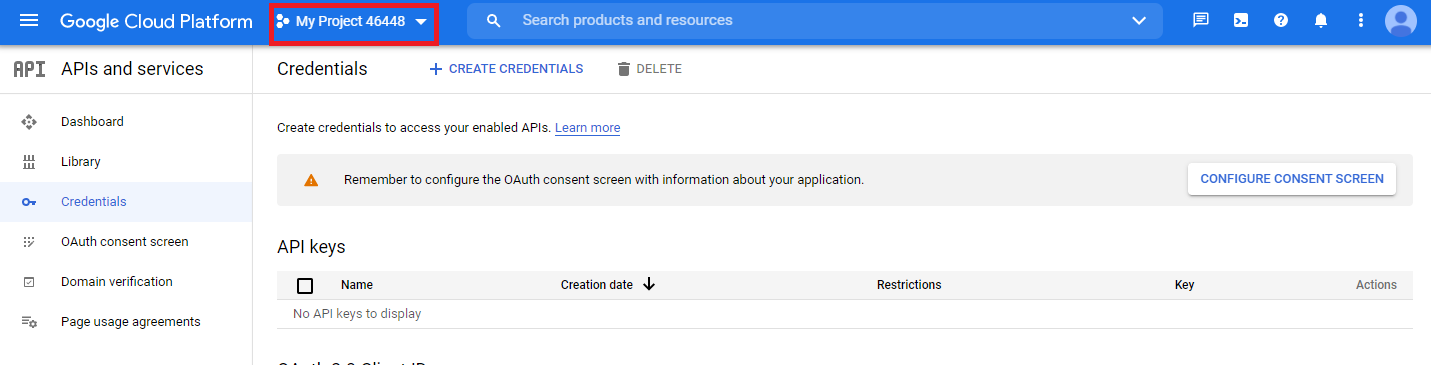
3. Click on the OAuth consent screen in the left sidebar. On this page you will select User Type as External and click on Create Button as shown on image below

After click on create button it will redirected to app registration page. You have to enter a project name, select user support email and enter your email id on the Developer Contact Information. And Click on SAVE AND CONTINUE button as shown on image below


5. Click on Credentials in the left sidebar and Click on CREATE CREDENTIALS. It will open up a popup, you will select OAuth client ID as shown on image below.

6.After click on OAuth client ID, it will redirected to Create OAuth Client ID Page. You have to select web application in Application Type Dropdown. Next, Enter the details Name, URIs as shown on image below and Save it.
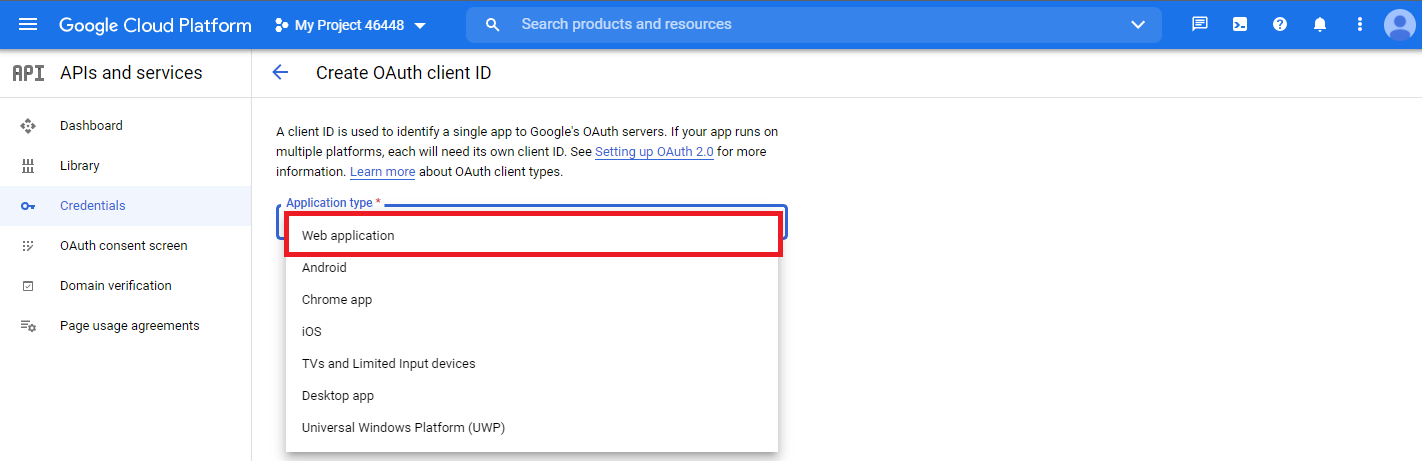


7.After click on SAVE button, it will redirected to Credentials Page. And, It will open up a popup with Client Id and Client Secret as shown on image below

Google API Client Library for PHP
You could download Google API php Client Library from the googleapis github. After download it, Extract the zip file and rename to google-api-php-client in sample folder.
Google API Client Library Integration With PHP
In this example we used four php files.
Files and Directory
sample/ ├── google-api-php-client/ ├── gc_config.php ├── login.php ├── home.php └── logout.php
<?php require "google-api-php-client/vendor/autoload.php"; $clientId="<CLIENT_ID>"; $clientSecret="<CLIENT_SECRET>"; $redirectURI="<REDIRECT_URI>"; $client=new Google_Client(); $client->setClientId($clientId); $client->setClientSecret($clientSecret); $client->setRedirectURI($redirectURI); $client->addScope("email"); $client->addScope("profile"); ?>
<?php session_start(); include "gc_config.php"; if(isset($_GET["code"])){ $token=$client->fetchAccessTokenWithAuthCode($_GET["code"]); $client->setAccessToken($token["access_token"]); $obj=new Google_Service_Oauth2($client); $data=$obj->userinfo->get(); if(!empty($data->email)&&!empty($data->name)){ //if you want to register user details, place mysql insert query here $_SESSION["email"]=$data->email; $_SESSION["name"]=$data->name; header("location:home.php"); } } ?> <html> <head> <title>Login With Google Account in PHP</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" integrity="sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm" crossorigin="anonymous"> <head> <body> <div class='container mt-5 text-center'> <h2>Login With Google Account in PHP</h2><br> <a href='<?php echo $client->createAuthUrl(); ?>' class='btn btn-danger'>Login With Google</a> </div> </body> </html>
<?php session_start(); if(!isset($_SESSION["email"])){ header("location:login_page.php"); } ?> <html> <head> <title>Home</title> </head> <body> <h1>Login Successfully. Welcome <?php echo $_SESSION["name"]; ?></h1> <a href='logout.php'>Logout</a> </body> </html>
<?php session_start(); unset($_SESSION["name"]); unset($_SESSION["email"]); session_destroy(); header("location:login_page.php"); ?>
