Get Data from Ajax Post in Flask
This example shows, how to load second dropdown when first dropdown onchange using jQuery Ajax in Flask.
Output:
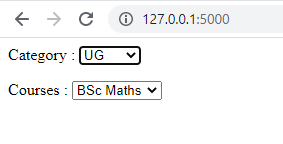
app.py
from flask import Flask,render_template,request app=Flask(__name__) @app.route("/") def index(): return render_template("index.html") @app.route("/getDataAjax",methods=['GET','POST']) def getDataAjax(): res=[] if request.method=='POST': courses={ 'ug':['BSc Maths','BSc CS','BA Tamil','BA English'], 'pg':['MSc Maths','MSc CS','MA Tamil','MA English'], } res=courses[request.form['data']] return render_template("get_data_ajax.html",data=res) if __name__=='__main__': app.run(debug=True)
index.html
<html> <head> <title></title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <p> Category : <select id='course_cate'> <option value=''>Select</option> <option value='ug'>UG</option> <option value='pg'>PG</option> </select> </p> <p> Courses : <select id='courses'> </select> </p> <script> $(document).ready(function(){ $("#course_cate").change(function(){ let cate=$(this).val(); if (cate!=''){ $.ajax({ url:"{{url_for('getDataAjax')}}", type:"POST", data:{"data":cate}, success:function(res){ $("#courses").html(res); } }); }else{ $("#courses").html(""); } }); }); </script> </body> </html>
get_data_ajax.html
{% for course in data %} <option value='{{course}}'>{{course}}</option> {% endfor %}
Run the Project:
- Run 'app.py' file.
- Browse the URL 'localhost:5000'