File Upload with Ajax in Python Flask
The following example shows, how to upload a file using jquery ajax in Python Flask.
File Structure:
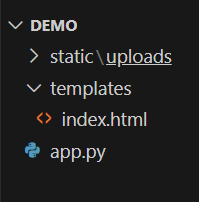
- Create the 'uploads' folder inside of 'static' folder for file uploading.
- Add the following code in app.py to create a basic Flask application
app.py
# app.py from flask import Flask, request, jsonify, render_template import os from werkzeug.utils import secure_filename app = Flask(__name__) app.config['UPLOAD_FOLDER'] = 'static/uploads' ALLOWED_EXTENSIONS = {'png', 'jpg', 'jpeg', 'gif'} # Ensure upload folder exists os.makedirs(app.config['UPLOAD_FOLDER'], exist_ok=True) def allowed_file(filename): return '.' in filename and filename.rsplit('.', 1)[1].lower() in ALLOWED_EXTENSIONS @app.route('/') def index(): return render_template('index.html') @app.route('/upload', methods=['POST']) def upload_file(): if 'file' not in request.files: return jsonify({'error': 'No file part'},status=400) file = request.files['file'] if file.filename == '': return jsonify({'error': 'No selected file'},status=400) if file and allowed_file(file.filename): filename = secure_filename(file.filename) file_path = os.path.join(app.config['UPLOAD_FOLDER'], filename) file.save(file_path) return jsonify({ 'message': 'File uploaded successfully', 'filename': filename, 'url': f'/static/uploads/{filename}' }) return jsonify({'error': 'File type not allowed'},status=400) if __name__ == '__main__': app.run(debug=True)
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Image Upload</title> <link href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap/5.3.0/css/bootstrap.min.css" rel="stylesheet"> <style> .preview-container { max-width: 300px; margin: 20px 0; } .preview-image { max-width: 100%; display: none; } #progress-bar { display: none; } </style> </head> <body> <div class="container mt-5"> <h2>Image Upload</h2> <form id="upload-form"> <div class="mb-3"> <label for="file-input" class="form-label">Choose Image</label> <input type="file" class="form-control" id="file-input" accept="image/*"> </div> <button type="submit" class="btn btn-primary">Upload</button> </form> <div class="progress mt-3" id="progress-bar"> <div class="progress-bar" role="progressbar" style="width: 0%"></div> </div> <div class="preview-container"> <img id="preview-image" class="preview-image" > </div> <div id="message"></div> </div> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <script> $(document).ready(function() { // Preview image before upload $('#file-input').change(function() { const file = this.files[0]; if (file) { const reader = new FileReader(); reader.onload = function(e) { $('#preview-image').attr('src', e.target.result).show(); } reader.readAsDataURL(file); } }); // Handle form submission $('#upload-form').submit(function(e) { e.preventDefault(); const fileInput = $('#file-input')[0]; if (!fileInput.files.length) { $("#message").text("Please select a file"); return; } const formData = new FormData(); formData.append('file', fileInput.files[0]); $('#progress-bar').show(); $('.progress-bar').css('width', '0%'); $.ajax({ url: '/upload', type: 'POST', data: formData, processData: false, contentType: false, xhr: function() { const xhr = new window.XMLHttpRequest(); xhr.upload.addEventListener('progress', function(e) { if (e.lengthComputable) { const percent = (e.loaded / e.total) * 100; $('.progress-bar').css('width', percent + '%'); } }, false); return xhr; }, success: function(response) { $("#message").text("File Upload Successfully "); }, error: function(xhr) { const response = xhr.responseJSON || {}; $("#message").text("File Upload Failed "); }, complete: function() { setTimeout(function() { $('#progress-bar').hide(); $('#message').hide(); }, 1000); } }); }); }); </script> </body> </html>
Run the Project:
- Run 'app.py' file.
- Browse the URL 'localhost:5000/fileUpload'
Output:
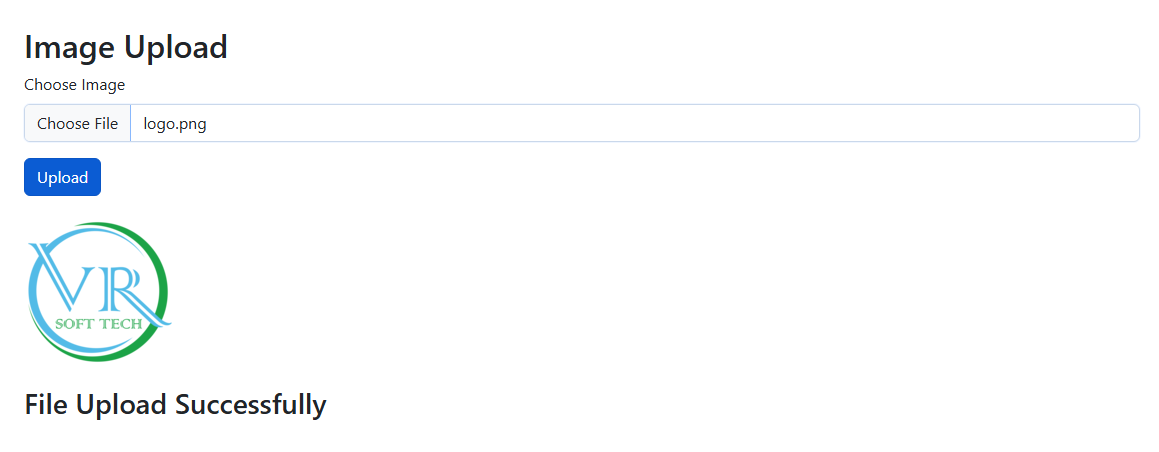