Django Link Static File
In this tutorial we see how to Link Static File in Django. Static files (CSS, JavaScript, images) are an essential part of web applications. Django provides a built-in way to manage and serve these files efficiently.
Files & Folder Structure
Here is the project folder structure and files.
Set Up Static Files Configuration
In myproject/settings.py, make sure the following settings are properly configured
# Static files (CSS, JavaScript, Images) STATIC_URL = '/static/' # URL prefix for static files # Directory where static files are collected during deployment STATIC_ROOT = os.path.join(BASE_DIR, 'staticfiles') # Additional directories for static files STATICFILES_DIRS = [ os.path.join(BASE_DIR, 'static'), # Your app-specific static files ]
Link Static Files in Templates
- Load the static template tag at the beginning of your HTML file.
- Use {% load static %} to reference the static file path.
This Django template links static files using {% load static %}, including CSS for styling, an image for display, and JavaScript for functionality.
{% load static %} <html> <head> <title>Link Static File in Django</title> <!-- Link CSS file --> <link rel="stylesheet" href="{% static 'css/style.css' %}"> </head> <body> <h1>Welcome to Website</h1> <!-- Link Image file --> <img src="{% static 'images/bg.jpg' %}"> <!-- Link JavaScript file --> <script src="{% static 'js/script.js' %}"></script> </body> </html>
This CSS applies a light gray background, uses Arial for text, and centers content for a clean and readable design.
body { background-color: #f0f0f0; font-family: Arial, sans-serif; text-align: center; }
This JavaScript code triggers an alert when the DOM is fully loaded, ensuring it runs only after the page content is ready.
document.addEventListener("DOMContentLoaded", function() { alert("JavaScript file loaded successfully!"); });
This Django configuration maps the root URL to myapp.urls, allowing the app to manage its own routing.
from django.contrib import admin from django.urls import path,include urlpatterns = [ path('',include('myapp.urls')) ]
This Django view function renders and returns the index.html template when the index route is accessed.
from django.shortcuts import render def index(request): return render(request,"index.html")
This Django URL configuration redirects the root path to index and maps /index to its corresponding view in views.py.
from django.urls import path from django.views.generic import RedirectView from .import views urlpatterns = [ path('', RedirectView.as_view(pattern_name='index', permanent=False)), #Redirect to index path('index',views.index,name="index"), ]
Output
This output displays a styled welcome message with a light gray background, and shows an alert saying "JavaScript file loaded successfully!" when the page loads.
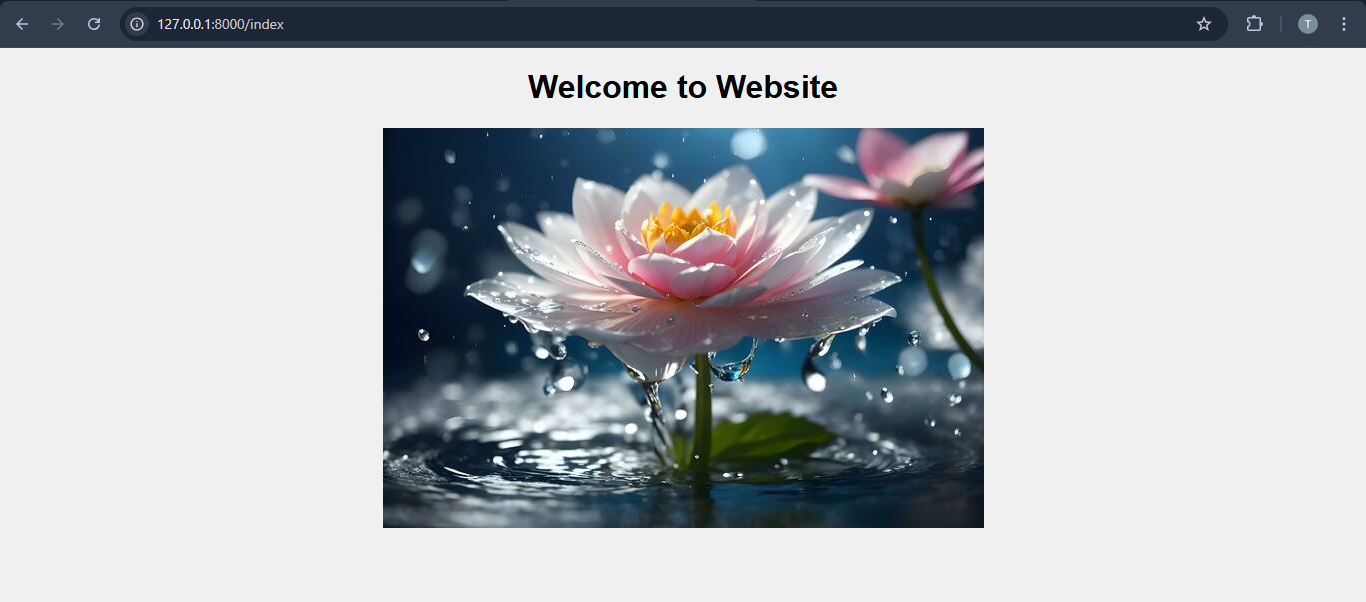