How to Show Html Content in Flutter
In this example, you will learn to show HTML content in Flutter using flutter_html package.
1. Adding flutter_html
package to your pubspec.yaml
file.
Open the pubspec.yaml file and find dependencies. Add flutter_html: ^1.3.0 as shown as image.
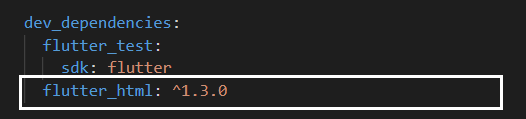
2. Import flutter_html
import 'package:flutter_html/flutter_html.dart';
3. Use Html
widget to display html content
Container( child: Html( data:"<h1 style='color:red;'><u> Hello World </u></h1>", ), ),
Example
sample.dart
import 'package:flutter/material.dart'; import 'package:flutter_html/flutter_html.dart'; class Example extends StatelessWidget { final String htmlcontent = """ <h1>Hello </h1> <h2 style='color:red'>World</h2> <p>This is sample paragraph</p> <p> H<sup>2</sup>O </p> <p> A<sub>2</sub> </p> <i>italic</i> <b>bold</b> <u>underline</u> <s>strike </s> <ul> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul> <br><br> <img src="https://vrsofttech.com/flutter-tutorials/images/vr.png" width="100"> """; @override Widget build(BuildContext context) { return SafeArea( child: Scaffold( body: Column( children: [ Container( child: Html( data: htmlcontent, ), ), ], ), ), ); } }
Output :
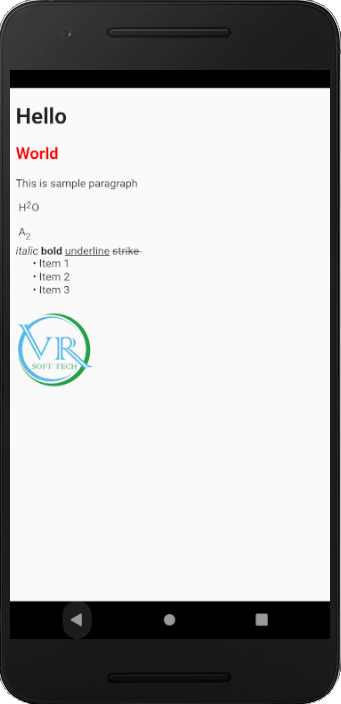