Flutter Text Widget
In Flutter, a Text widget is used to display a text with a single line or multiple lines.
Create a Text Widget
Syntex
Text("This is sample text")
Styling a Text widget
We can style a Text widget using the style property. The style property that takes a TextStyle object used to change the appearance of the text, such as the color, fontstyle,fontsize, fontweight, line-height etc
Here's an example of how to style a Text widget in Flutter:
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( body: Center( child: Text( 'There are many variations of passages of Lorem Ipsum available, but the majority have suffered alteration in some form', style: TextStyle( fontSize: 20, fontWeight: FontWeight.bold, fontStyle: FontStyle.italic, color: Colors.white, letterSpacing: 2, wordSpacing: 10, height: 2, //line height decoration: TextDecoration.underline, decorationColor: Colors.green, decorationThickness: 1.0, backgroundColor: Colors.purple, ), ), ), ), ); } }
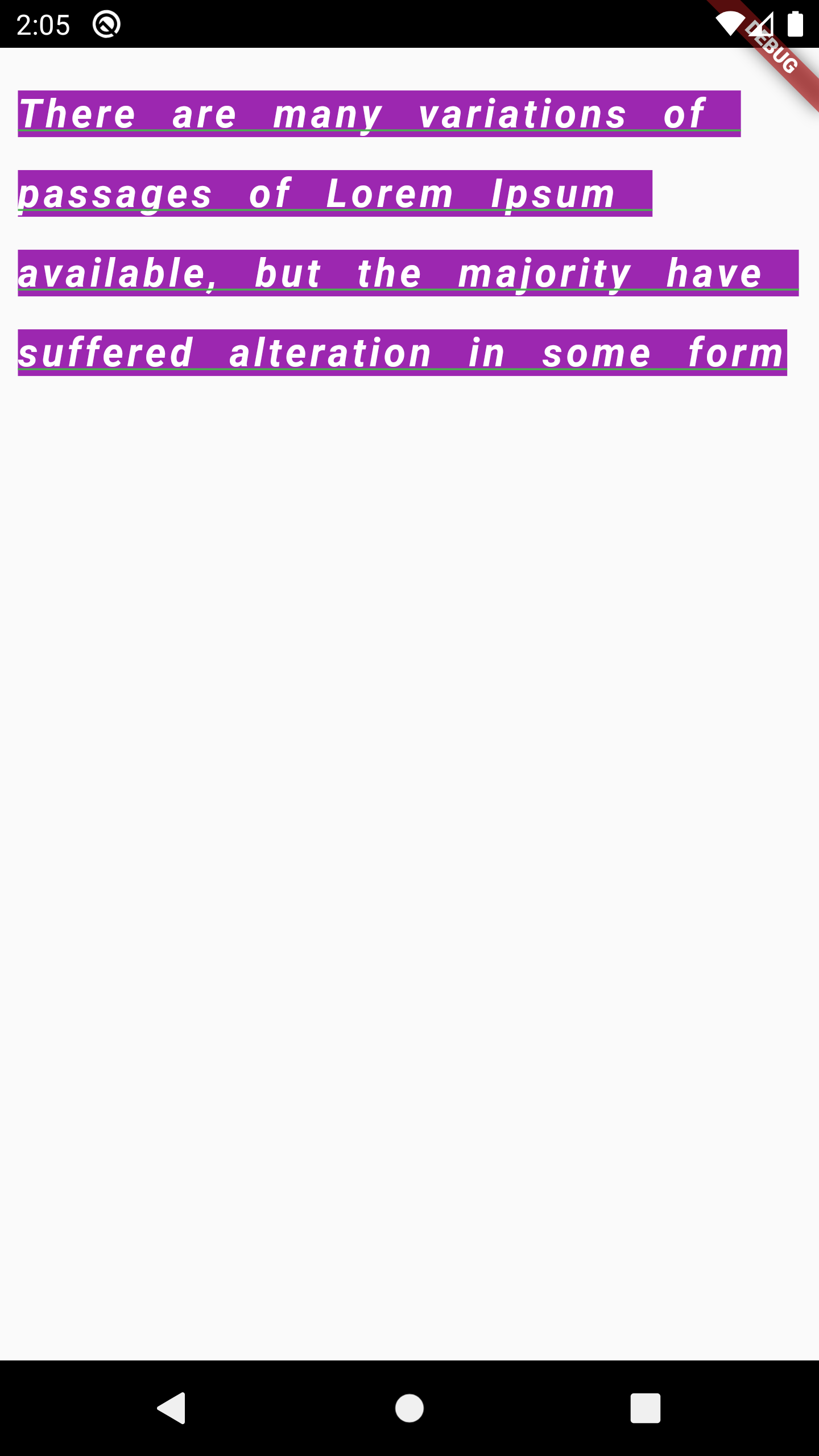
Align Text in Text Widget
we can align text within a Text widget using the textAlign property.
Here's an example:
Text( "Align Text in Flutter", textAlign: TextAlign.center, )
Overflow Text in Text Widget
We can handle text overflow using the overflow property with maxLines
Here's an example:
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class Demo extends StatelessWidget { @override Widget build(BuildContext context) { return SafeArea( child: Scaffold( body: Padding( padding: const EdgeInsets.all(8.0), child: Column( mainAxisAlignment: MainAxisAlignment.start, children: [ Text( "There are many variations of passages of Lorem Ipsum available, but the majority have suffered alteration in some form", textAlign: TextAlign.justify maxLines: 3, //Set the maximum number of lines overflow: TextOverflow.ellipsis, ), Divider(), Text( "There are many variations of passages of Lorem Ipsum available, but the majority have suffered alteration in some form", textAlign: TextAlign.justify, maxLines: 3, //Set the maximum number of lines overflow: TextOverflow.clip, ), Divider(), Text( "There are many variations of passages of Lorem Ipsum available, but the majority have suffered alteration in some form", textAlign: TextAlign.justify, maxLines: 3, //Set the maximum number of lines overflow: TextOverflow.fade, ), ], ), ), ), ); } }
