FPDF - Table
In this example, we will learn how to create a table in a PDF with PHP using FPDF. There is no inbuild function to create table in FPDF. We have to create a table using collection of Cells.
The following example shows, how to create a diffrent type of tables in FPDF.
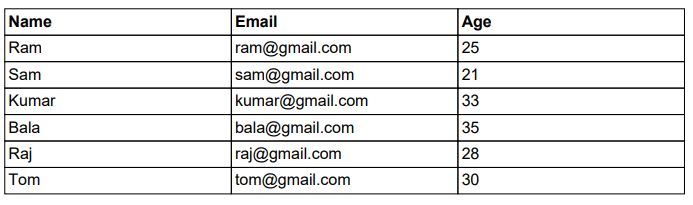
Example 1
<?php require('fpdf/fpdf.php'); class PDF extends FPDF { function createTable($header,$data){ $this->SetFont('Arial','B',12); foreach($header as $h){ $this->Cell(60,7,$h,1); } $this->Ln(); $this->SetFont('Arial','',12); foreach($data as $row){ foreach($row as $col){ $this->Cell(60,7,$col,1); } $this->Ln(); } } } $pdf=new PDF(); $pdf->AddPage(); //Table Header $header=["Name","Email","Age"]; //Table Rows $data=[ ["Ram","ram@gmail.com",25], ["Sam","sam@gmail.com",21], ["Kumar","kumar@gmail.com",33], ["Bala","bala@gmail.com",35], ["Raj","raj@gmail.com",28], ["Tom","tom@gmail.com",30], ]; $pdf->createTable($header,$data); $pdf->Output(); ?>
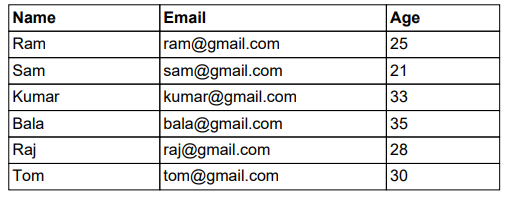
Example 2
<?php require('fpdf/fpdf.php'); class PDF extends FPDF { function createTable($header,$data){ $width=[40,60,30]; $this->SetFont('Arial','B',12); for($i=0;$i<count($header);$i++){ $this->Cell($width[$i],7,$header[$i],1); } $this->Ln(); $this->SetFont('Arial','',12); foreach($data as $row){ $this->Cell($width[0],7,$row[0],1,0); $this->Cell($width[1],7,$row[1],1,0); $this->Cell($width[2],7,$row[2],1,0); $this->Ln(); } } } $pdf=new PDF(); $pdf->AddPage(); //Table Header $header=["Name","Email","Age"]; //Table Rows $data=[ ["Ram","ram@gmail.com",25], ["Sam","sam@gmail.com",21], ["Kumar","kumar@gmail.com",33], ["Bala","bala@gmail.com",35], ["Raj","raj@gmail.com",28], ["Tom","tom@gmail.com",30], ]; $pdf->createTable($header,$data); $pdf->Output(); ?>
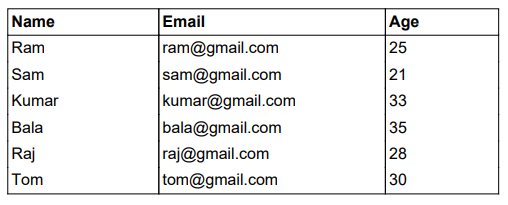
Example 3
<?php require('fpdf/fpdf.php'); class PDF extends FPDF { function createTable($header,$data){ $width=[40,60,30]; $this->SetFont('Arial','B',12); for($i=0;$i<count($header);$i++){ $this->Cell($width[$i],7,$header[$i],1); } $this->Ln(); $this->SetFont('Arial','',12); foreach($data as $row){ $this->Cell($width[0],7,$row[0],'LR'); $this->Cell($width[1],7,$row[1],'LR'); $this->Cell($width[2],7,$row[2],'LR'); $this->Ln(); } $this->Cell(array_sum($width),0,'','T'); } } $pdf=new PDF(); $pdf->AddPage(); //Table Header $header=["Name","Email","Age"]; //Table Rows $data=[ ["Ram","ram@gmail.com",25], ["Sam","sam@gmail.com",21], ["Kumar","kumar@gmail.com",33], ["Bala","bala@gmail.com",35], ["Raj","raj@gmail.com",28], ["Tom","tom@gmail.com",30], ]; $pdf->createTable($header,$data); $pdf->Output(); ?>
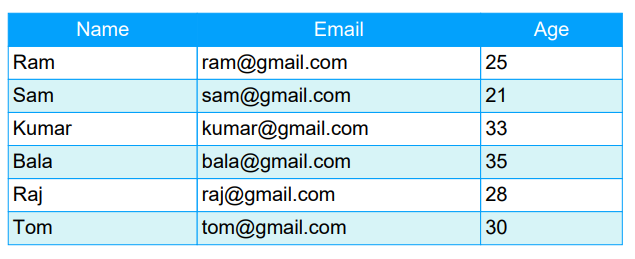
Example 4
<?php require('fpdf/fpdf.php'); class PDF extends FPDF { function createTable($header,$data){ $width=[40,60,30]; $this->SetFillColor(3,161,252); $this->SetTextColor(255,255,255); $this->SetDrawColor(3,161,252); for($i=0;$i<count($header);$i++) { $this->Cell($width[$i],7,$header[$i],1,0,'C',true); } $this->Ln(); $this->SetFillColor(215,244,247); $this->SetTextColor(0,0,0); $fill=false; foreach($data as $row){ $this->Cell($width[0],7,$row[0],1,0,'L',$fill); $this->Cell($width[1],7,$row[1],1,0,'L',$fill); $this->Cell($width[2],7,$row[2],1,0,'L',$fill); $this->Ln(); $fill=!$fill; } } } $pdf=new PDF(); $pdf->AddPage(); $pdf->SetFont('Arial','',12); //Table Header $header=["Name","Email","Age"]; //Table Rows $data=[ ["Ram","ram@gmail.com",25], ["Sam","sam@gmail.com",21], ["Kumar","kumar@gmail.com",33], ["Bala","bala@gmail.com",35], ["Raj","raj@gmail.com",28], ["Tom","tom@gmail.com",30], ]; $pdf->createTable($header,$data); $pdf->Output(); ?>